Why and How to Use Laravel Strict Mode in Your Projects?
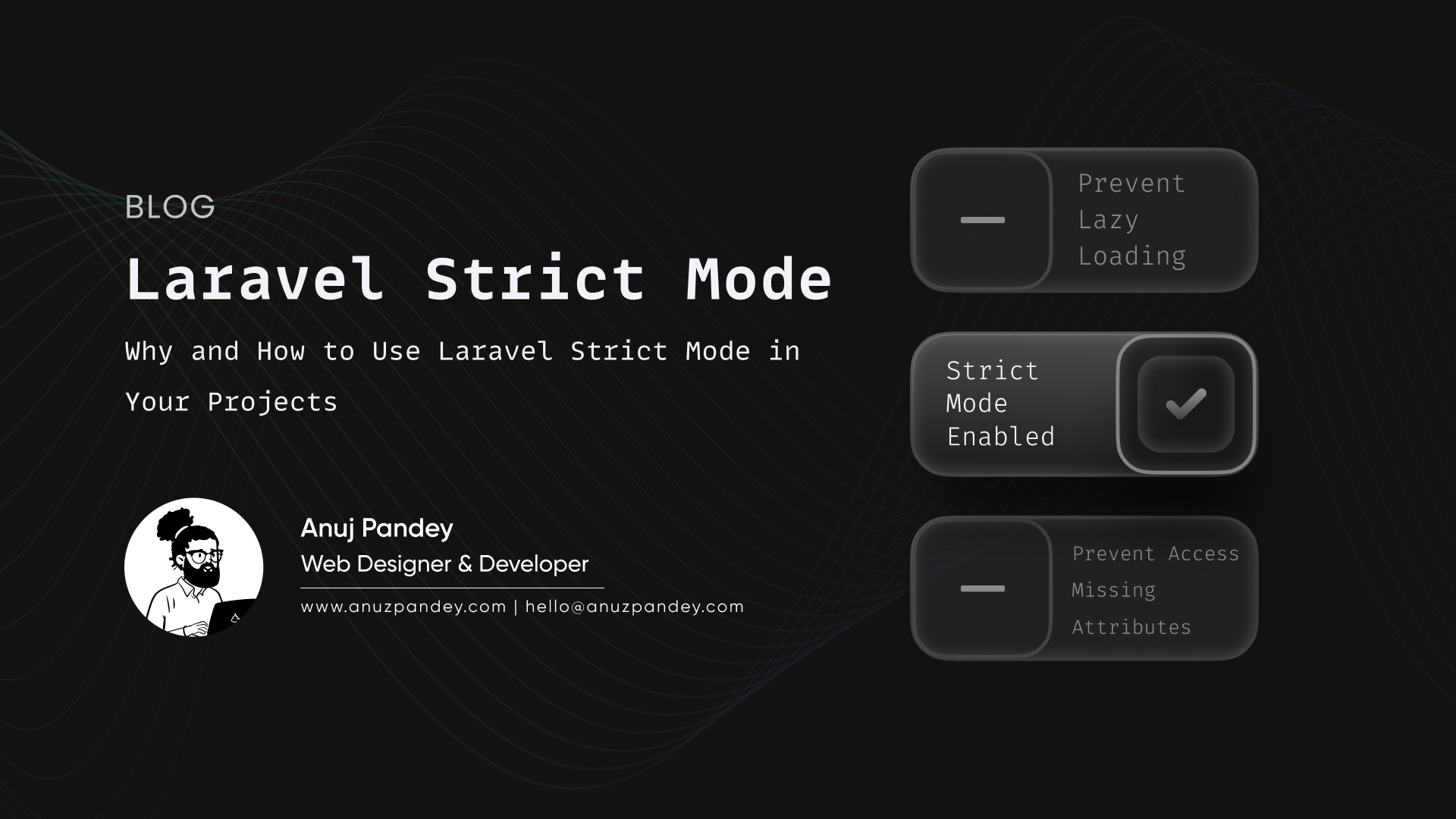
Laravel is a robust PHP framework that offers a lot of power to developers. In this article, we’ll explore how to use Laravel Strict Mode in our application and how it can benefit you.
Prevent N+1 Query Problem with Lazy Loading
You might have heard of Lazy Loading before. Simply put, it's when you retrieve a parent model along with its related child models. For example, imagine you have a User model and each user has a role. Here's how that looks in Laravel:
To get the roles for a specific post, we'd do something like this:
And in the view, we would loop through the roles like this:
Here, we introduced the N+1 query problem. This means that for each user, we are making an additional query to fetch its role. This can be a performance bottleneck when you have a large number of users. And if we look at the query log, we can see that we are making N+1 queries:
So if you have 1000 users, our application will run 1001 queries, which is not good for performance. So, to prevent this, we leverage Laravel's Eager Loading. Instead of loading the roles for each user individually, we can load all the roles in a single query.
Now, if we look at the query log, we can see that we are making only 2 queries:
But as a developer, it's easy to forget to use eager loading. And that's where Laravel's Strict Mode comes in. To help us remember and avoid performance issue, Laravel introduces Strict Mode.
How to Enable Strict Mode
Open the AppServiceProvider.php file and add the following like in the boot method:
The ! $this->app->isProduction() part ensures that Laravel only throws warnings about Lazy Loading during the development environment. This way, we can catch the issue early and fix it before deploying to production.
With this in place, if we forget to use eager loading, Laravel will throw a warning like this:
Prevent Silently Ignored Attributes
Another feature of Strict Mode is Preventing Silently Discarding Attributes. Let’s break this down.
Say, for example, we had a User model, and later we decided to add a new column called username to the users table. But we forgot to update the User model $fillable property.
And in our UserController we have the following code:
Now here, we will get the integrity constraint violation error because we're trying to insert value into the username column which is not allowed. But the exception is not so helpful in this case, so we can use the Model::preventSilentlyDiscardingAttributes() method in the AppServiceProvider to prevent silently discarding attributes.
With this we will get the MassAssignmentException exception with a helpful message:
Prevent Accessing Non-Existing Attributes
Sometimes, we might accidentally access an attribute that doesn't exist in our model or make a typo. In such cases, Laravel will silently return null without throwing any error. This can be a source of bugs that are hard to track down.
Example
To prevent this, we can use the Model::preventAccessingMissingAttributes() method in the AppServiceProvider:
Now, if we try to access a non-existing attribute, Laravel will throw UnknownAttributeException:
Enabling All Strict Features Together
If we want to turn all three Strict Mode features on, we can do so by adding the following code in the AppServiceProvider:
This will activate all the three strict checks, ensuring our application is more reliable and free from common mistakes.
Conclusion
In this article, we’ve covered the three main features of Laravel’s Strict Mode and how to implement them in your projects:
- Prevent Lazy Loading: Avoids inefficient database queries.
- Prevent Silently Discarding Attributes: Ensures all model attributes are accounted for.
- Prevent Accessing Missing Attributes: Stops accessing undefined model properties.
- Enabling All Strict Features Together: Activates all three strict checks.Ò
Your feedback is valuable to me, so if you'd like to see more content like this in the future, please don't hesitate to let me know.
Keep coding, keep exploring, and keep inspiring. 🐼